איך להעלות תוך כמה שעות תוכנות פייתון לכל העולם בחינם
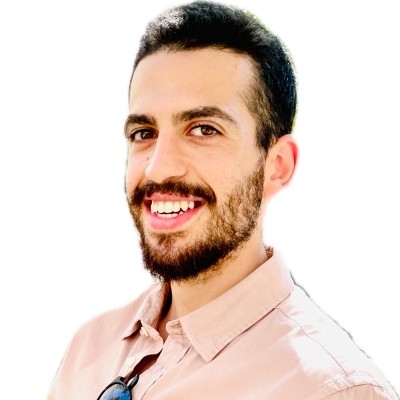
מאז שישי האחרון העלתי לרשת כבר יותר משבע תוכנות שמאות משתמשים ניסו. למשל, התוכנה שאתם רואים בסרטון זמינה מהטלפון או מהמחשב שלכם בקישור הבא: dorpascal.com/8-queens
חיפשתי דרך לעשות את זה עם מעט ידע בפרונט, בלי לכתוב מחדש בסיסי קוד, ובלי לשלם על שרתים. מצאתי פתרון וכתבתי לכם אותו כמדריך מקוצר שלא מצאתי בשום מקום ברשת. אלה הצעדים בתיאור כללי, וצריך בשבילם ידע מקדים בפייתון. לפרטים נוספים אתם מוזמנים לקרוא את קבצי המקור שצירפתי באתר של כל תוכנה.
- תשיגו דומיין חינמי
סטודנטים יכולים לקבל דומיין כמו dorpascal.com דרך Namecheap. תגדירו אותו לפי המפרט של GitHub Pages או שתשתמשו ב- itch.io, שזאת פלטפורמה למפתחי אינדי. למשל, https://dor-sketch.itch.io - תבנו ממשק משתמש עם Pygame
זאת ספריה חינמית למפתחי משחקים, אבל לא רק. תתאימו את הקוד לריצה ברשת בעזרת הספרייהasyncio
: תהפכו פונקציות שאחראיות על קלט פלט ל-async
דרך הוספת המילה הזאת להגדרה שלהן, ותשתמשו ב-await
בקריאות אליהן. - לבסיסי נתונים - תפתחו פרויקט ב-Firebase
זאת פלטפורמת backend של גוגל. תפתחו חשבון, תגדירו פרויקט חדש, ותצרו Firestore Database (חינמי בסקייל קטן, להבנתי). משם תגדירו את הכללים של בסיס הנתונים שלכם דרך ה-API
לניהול התקשורת בהמשך. - תארזו את הקוד כ-
WebAssembly
בעזרת PygBag
תריצוpygbag <project dir>
, ותראו שני קבצים שנוצרים ממנה: index.html ו-apk
. בשלב הזה אתם כבר יכולים להשתמש בתוכנה מתוך הדפדפן שלכם בבית. אתם גם יכולים להריץ עליה טסטים דרךlocalhost
. - בשביל לחבר את התוכנה לבסיס הנתונים תשתמשו xn---platform-4dl.window(
<js>
) כדי לקרוא ולכתוב ל-local storage
. מחוץ לתוכנה, תאזינו למשתנים שאתם רוצים, ותוסיפו סקריפטים לשאילתות. שימו לב שערוץ התקשורת חשוף למשתמשים, ושהפתרון הזה לא מתאים לבסיסי נתונים עם מידע רגיש. - עלו לאוויר
תריצוpygbag --archive
ותשמרו את הקובץ שנוצר ב-GitHub Pages או ב- itch.io.
Published on LinkedIn on June 13, 2024